Editorial for An Animal Contest 7 P5 - Squirrel Scheming
Submitting an official solution before solving the problem yourself is a bannable offence.
Author:
Hint 1
Consider the following problem: Given an arbitrarily rooted tree, what is the minimum distance of a path that starts from the root, visits every single node once then returns to the root?
Answer
The answer is where
is the number of edges in the tree. We traverse each edge once when entering a subtree, and another time when leaving the subtree to return to the root. Overall, we traverse each edge twice so the answer is
.
Hint 2
If we don't consider the lexicographic part of the problem, this problem is the exact same as the above except we don't need to return to the root. Think about the maximum distance we can "save" from not returning to the root.
Answer
The maximum distance we can "save" is equal to the diameter of the tree. Which means the minimum cost path is equal to where
is the number of edges, and
is the diameter of the tree.
Hint 3
Find the optimal starting node for a permutation, then build the rest of the permutation.
Subtask 1
Let's first find the optimal starting node in the permutation. This node is simply the minimum node that is an endpoint of any diameter in the tree. To find this node, one can simply run a DP on the tree. Let's call this node .
Next, observe that for any diameter , there is a permutation where
is the last node and achieves the minimum cost. This is because after we visit
we do not need to return to
thus saving a cost equal to the length of the diameter of the tree, which is the maximum amount you can save.
This encourages a solution that first fixes the start node and the end node, then constructs the rest of the permutation. As it turns out, knowing the first and last node of the permutation makes the problem a lot simpler.
To get an idea of what the algorithm looks like, consider the following tree:
Diagram
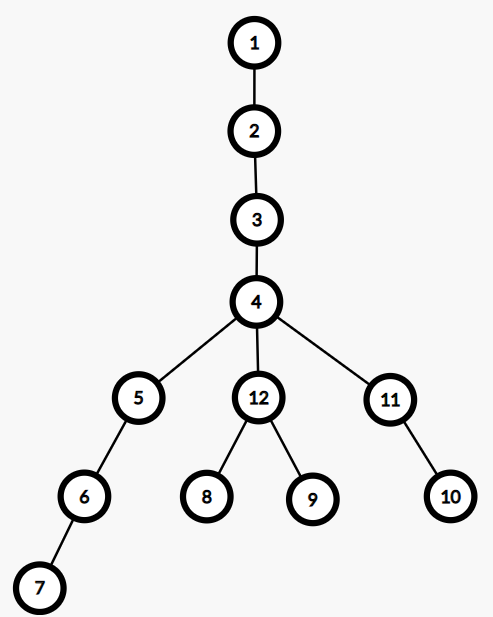
Clearly, the optimal starting node is
Time Complexity:
Subtask 2
Since there are diameters in the worst case, we can no longer afford to run the algorithm directly for each diameter. To optimize, instead of fixing the diameter, we can actually make our sorting slightly more sophisticated to find an optimal diameter. As with the previous algorithm, sort the nodes by the minimum node present in each subtree. However, this time also keep track of which subtrees contain at least one ending of a diameter. Now, instead of fixing the diameter, we can simply process the maximum value subtree that contains at least
diameter last.
Time Complexity:
Comments