Editorial for COCI '11 Contest 3 #6 Traka
Submitting an official solution before solving the problem yourself is a bannable offence.
Let's formally define the condition from the task that says that no car can wait with none of the workers. Let's denote some car and some worker
. By the time worker
is done with car
, worker
must be done with car
. Note that worker
can finish car
before this. If car
is sent
minutes after car
, the following must hold:
In other words, the time that it takes car to pass through all the workers up to and including
must be at least the time that takes car
to go through workers up to and including
.
If we denote by sum of
for workers through
, than minimum
for cars
and
can be written as:
From this, we can derive the brute-force solution. For each , we go through all the workers to find the maximum value
, and we sum up these maximum values with
. This solution has a complexity of
.
We can come up with a faster solution by taking a look at the expression that we must maximize. Since is fixed and all the factors are positive we can divide this expression by
. If we denote this quotient
with
, we get
, i.e. a line equation. For each
that satisfies mentioned limits, we get a line in the plane. From these lines, we must find the one that gives the maximum value for some
.
This idea is often useful in algorithmic competitions, so we will describe it here in the general case. Let's take a look at how this maximum of lines looks like.
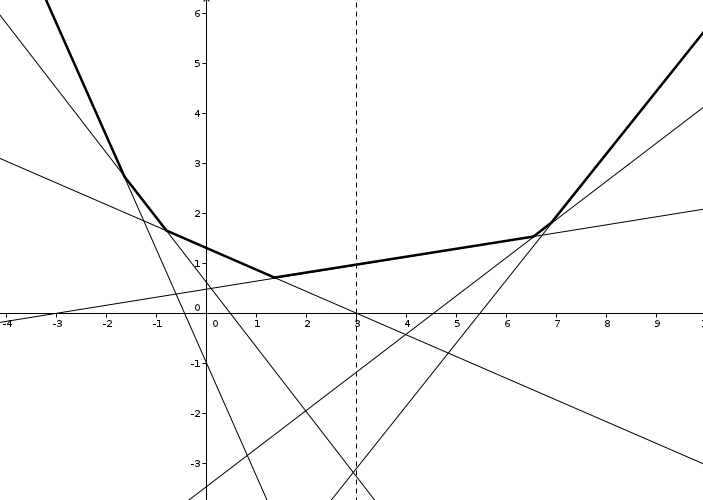
It's a polygonal line enclosing a convex region of the plane. Lines that are part of this line are sorted in increasing order of their slopes. Also, no line is above the previous two lines. This leads us to the following algorithm.
We sort the lines in increasing order of slopes. We push the first two to the stack, and proceed to traverse the rest of the lines. When processing the next line in order, we pop the top line of the stack, as long as the intersection of the top two lines on the stack is below the current line. When this is no longer true, we push the current line to the stack.
We denote the line that's pushed on the stack by
.
We can find the maximum value for some by using binary search, since
is monotonic with respect to
.
The complexity of this approach is , or to be more precise,
where
is the number of lines that are part of the convex boundary.
There is also an alternative solution that represents the expression we are trying to maximize using dot product, and uses convex hulls.
Comments