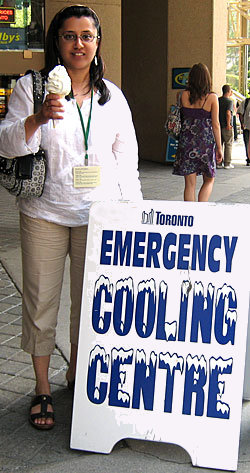
Jack and Jill play a game called Hotter, Colder. Jill has a number between and
, and Jack makes repeated attempts to guess it.
Each of Jack's guesses is a number between and
. In response to each guess, Jill answers hotter, colder or same. For Jack's first guess, Jill answers same. For the remaining guesses Jill answers:
- hotter if this guess is closer to Jill's number than his previous guess
- colder if this guess is farther from Jill's number than his previous guess
- same if this guess is neither closer to nor further from Jill's number than his previous guess.
You are to implement a procedure HC(N) that plays Jack's role. This implementation may repeatedly call Guess(G), with a number between
and
. Guess(G) will return
to indicate hotter,
to indicate colder or
to indicate same. HC(N) must return Jill's number.
Example
For example, assume , and Jill has chosen the number
. When procedure HC makes the following sequence of calls to Guess, the results in the second column will be returned.
Call | Returned value | Explanation |
---|---|---|
Guess( |
Same (first call) | |
Guess( |
Hotter | |
Guess( |
Colder | |
Guess( |
Hotter | |
Guess( |
Same |
At this point, Jack knows the answer, and HC should return . It has taken Jack
guesses to determine Jill's number. You can do better.
Subtask 1 [25 points]
HC(N) must call Guess(G) at most times. There will be at most
calls to HC(N), with
between
and
.
Subtask 2 [25 points]
HC(N) must call Guess(G) at most times. There will be at most
calls to HC(N) with
between
and
.
Subtask 3 [25 points]
HC(N) must call Guess(G) at most times. There will be at most
calls to HC(N) with
between
and
.
Subtask 4 [up to 25 points]
Let be the largest integer, such that
. For this subtask your solution will score:
points, if HC(N) calls Guess(G)
times or more,
points, where
is the largest real number, such that
and HC(N) calls Guess(G) at most
times,
points, if HC(N) calls Guess(G) at most
times.
There will be at most calls to HC(N) with
between
and
.
Be sure to initialize any variables used by HC every time it is called.
Implementation Details
- Implementation folder:
/home/ioi2010-contestant/hottercolder/
(prototype: hottercolder.zip) - To be implemented by contestant:
hottercolder.c
orhottercolder.cpp
orhottercolder.pas
- Contestant interface:
hottercolder.h
orhottercolder.pas
- Grader interface:
grader.h
orgraderlib.pas
- Sample grader:
grader.c
orgrader.cpp
orgrader.pas
andgraderlib.pas
- Sample grader input:
grader.in.1
grader.in.2
.
Note: The input file contains several lines, each containingand Jill's number.
- Expected output for sample grader input: the grader will create files
grader.out.1
grader.out.2
etc.- If the implementation correctly implements Subtask
, one line of output will contain
OK 1
- If the implementation correctly implements Subtask
, one line of output will contain
OK 2
- If the implementation correctly implements Subtask
, one line of output will contain
OK 3
- If the implementation correctly implements Subtask
, one line of output will contain
OK 4 alpha α
- If the implementation correctly implements Subtask
Comments