Nagyerdő is a square-shaped forest located in the city of Debrecen, which can be modeled as an
grid of cells. The rows of the grid are numbered from
to
from north to south, and
the columns are numbered from
to
from west to east. We refer to the cell located at row
and column
of the grid as cell
.
In the forest, each cell is either empty or contains a tree. At least one cell in the forest is empty.
DVSC, the famous sports club of the city, is planning to build a new soccer stadium in the forest. A
stadium of size (where
) is a set of
distinct empty cells
. Formally
this means:
- for each
from
to
, inclusive, cell
is empty,
- for each
,
such that
, at least one of
and
holds.
Soccer is played using a ball that is moved around the cells of the stadium. A straight kick is defined to be either of the following two actions:
- Move the ball from cell
to cell
, where the stadium contains all cells between cell
and
in row
. Formally,
- if
then the stadium should contain cell
for each
such that
,
- if
then the stadium should contain cell
for each
such that
.
- if
Move the ball from cell
to cell
, where the stadium contains all cells between cell
and
in column
. Formally,
- if
then the stadium should contain cell
for each
such that
,
- if
then the stadium should contain cell
for each
such that
.
- if
A stadium is regular if it is possible to move the ball from any cell contained by the stadium to any
other cell contained by the stadium with at most straight kicks. Note that any stadium of size
is
regular.
For example, consider a forest of size , with cells
and
containing trees and every
other cell being empty. The figure below shows three possible stadiums. Cells with trees are
darkened, and cells contained by the stadium are striped.
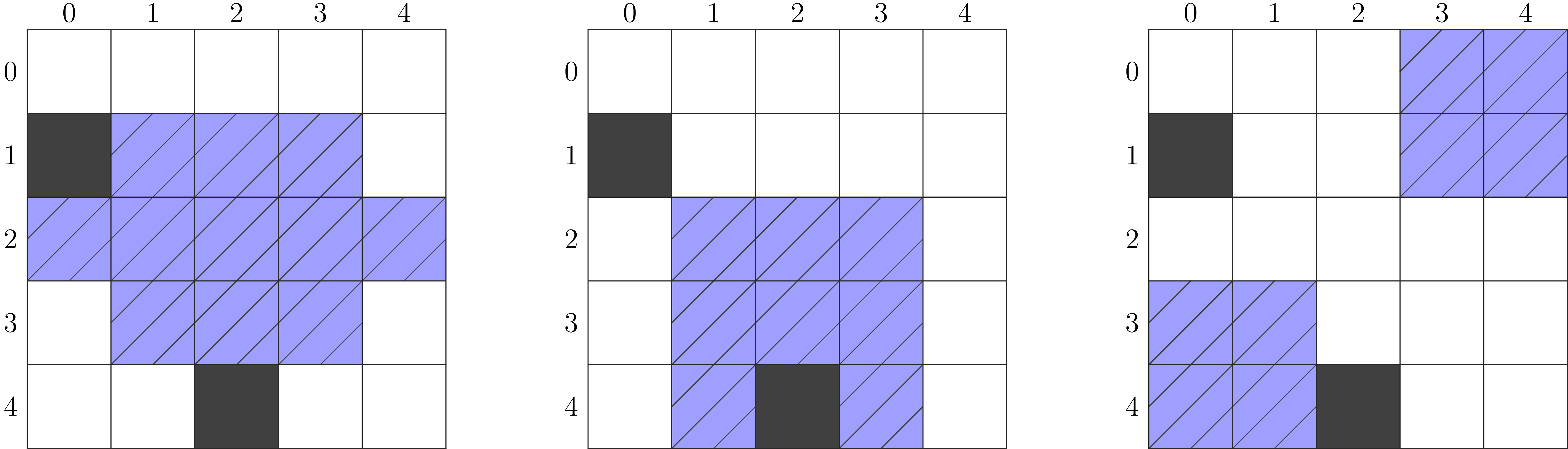
The stadium on the left is regular. However, the stadium in the middle is not regular, because at
least straight kicks are needed to move the ball from cell
to
. The stadium on the right
is also not regular, because it is impossible to move the ball from cell
to
using straight
kicks.
The sports club wants to build a regular stadium that is as big as possible. Your task is to find the
maximum value of such that there exists a regular stadium of size
in the forest.
Implementation Details
You should implement the following procedure.
int biggest_stadium(int N, std::vector<std::vector<int>> F)
: the size of the forest.
: an array of length
containing arrays of length
, describing cells in the forest. For each
and
such that
and
,
means that cell
is empty, and
means that it contains a tree.
- This procedure should return the maximum size of a regular stadium that can be built in the forest.
- This procedure is called exactly once for each test case.
Example
Consider the following call:
biggest_stadium(5, [[0, 0, 0, 0, 0],
[1, 0, 0, 0, 0],
[0, 0, 0, 0, 0],
[0, 0, 0, 0, 0],
[0, 0, 1, 0, 0]])
In this example, the forest is displayed on the left and a regular stadium of size is displayed on
the right of the following figure:
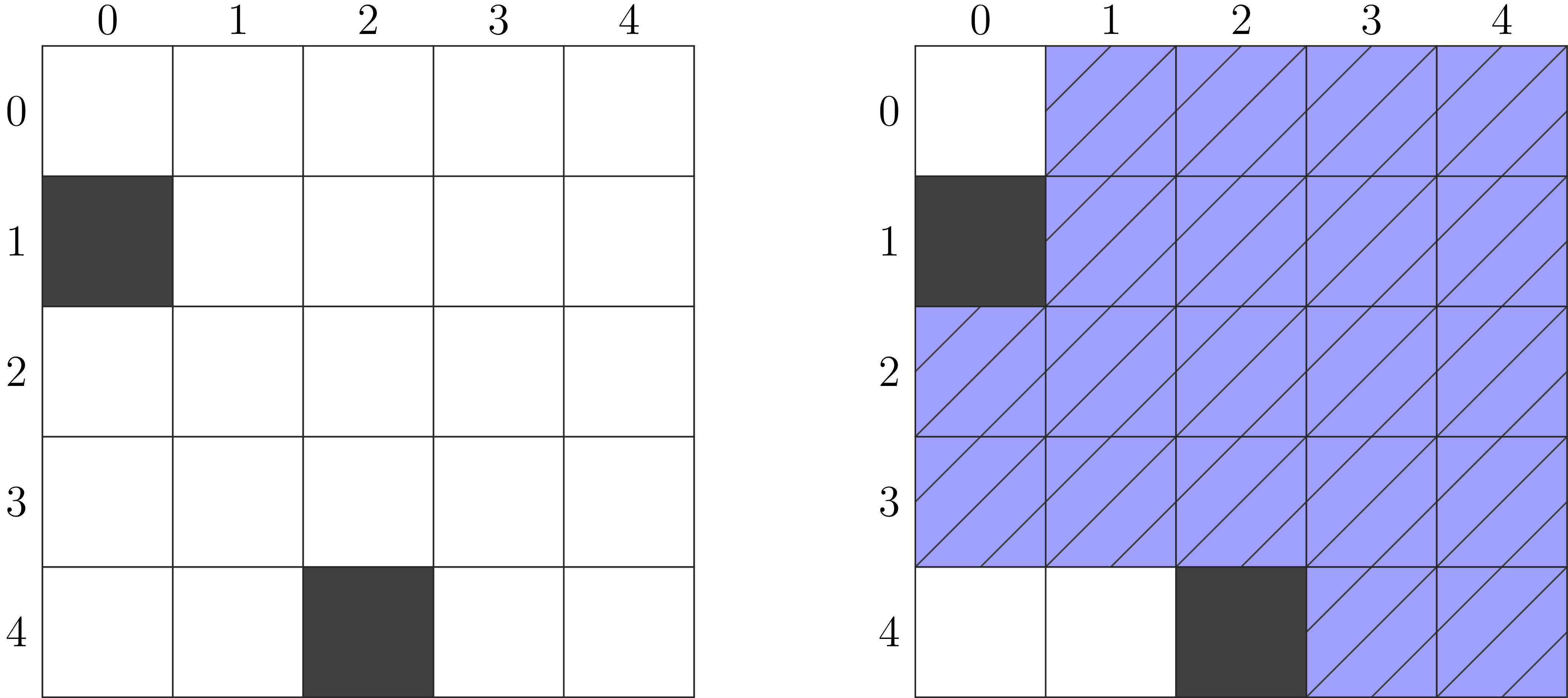
Since there is no regular stadium of size or greater, the procedure should return
.
Constraints
(for each
and
such that
and
)
- There is at least one empty cell in the forest. In other words,
for some
and
.
Subtasks
- (
points) There is at most one cell containing a tree.
- (
points)
- (
points)
- (
points)
- (
points)
- (
points) No additional constraints.
In each subtask, you can obtain of the subtask score if your program judges correctly whether
the set consisting of all the empty cells is a regular stadium.
More precisely, for each test case in which the set consisting of all the empty cells is a regular stadium, your solution:
- gets full points if it returns the correct answer (which is the size of the set consisting of all the empty cells).
- gets
points otherwise.
For each test case in which the set consisting of all the empty cells is not a regular stadium, your solution:
- gets full points if it returns the correct answer.
- gets
points if it returns the size of the set consisting of all the empty cells.
- gets
of the points if it returns any other value.
The score for each subtask is the minimum of the points for the test cases in the subtask.
Sample Grader
The sample grader reads the input in the following format:
- line
:
- line
:
The sample grader prints your answer in the following format:
- line
: the return value of
biggest_stadium
Attachment Package
The sample grader along with sample test cases are available here.
Comments