AI researchers at the University of Szeged are holding a robot programming contest. Your friend, Hanga, has decided to take part in the contest. The objective is to program the ultimate Pulibot, admiring the great intelligence of the famous Hungarian herding dog breed, the Puli.
Pulibot will be tested on a maze consisting of a grid of cells. The rows of the
grid are numbered from
to
from north to south and the columns of the grid are numbered
from
to
from west to east. We refer to the cell located at row
and column
of the grid
as cell
.
Consider a cell such that
and
. There are
cells adjacent to cell
:
- cell
is referred to as the cell west of cell
;
- cell
is referred to as the cell south of cell
;
- cell
is referred to as the cell east of cell
;
- cell
is referred to as the cell north of cell
.
Cell is called a boundary cell of the maze if
or
or
or
holds. Each
cell that is not a boundary cell of the maze is either an obstacle cell or an empty cell. Additionally,
each empty cell has a color, represented by a nonnegative integer between
and
, inclusive.
Initially, the color of each empty cell is
.
For example, consider a maze with and
, containing a single obstacle cell
:
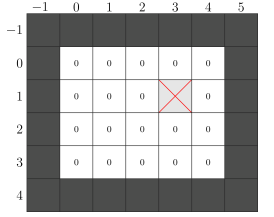
The only obstacle cell is denoted by a cross. Boundary cells of the maze are shaded. The number in each empty cell represents its color.
A path of length
from cell
to cell
is a sequence of pairwise distinct empty
cells
in which for each
the cells
and
are
adjacent.
Note that a path of length contains exactly
cells.
At the contest, the researchers set up a maze in which there exists at least one path from cell
to cell
. Note that this implies that cells
and
are guaranteed
to be empty.
Hanga does not know which cells of the maze are empty and which cells are obstacles.
Your task is to help Hanga to program Pulibot so that it is capable of finding a shortest path (that is,
a path of minimum length) from cell to cell
in the unknown maze set up by
the researchers. The specification of Pulibot and the rules of the contest are described below.
Note that the last section of this problem statement describes a display tool you can use to visualize Pulibot.
Pulibot's Specification
Define the state of a cell for each
and
as an integer so that:
- if cell
is a boundary cell then its state is
;
- if cell
is an obstacle cell then its state is
;
- if cell
is an empty cell then its state is the color of the cell.
Pulibot's program is executed as a sequence of steps. In each step, Pulibot recognizes the states of nearby cells and then performs an instruction. The instruction it performs is determined by the recognized states. A more precise description follows.
Suppose that at the beginning of the current step, Pulibot is at cell , which is an empty cell.
The step is performed as follows:
- First, Pulibot recognizes the current state array, that is, the array
, consisting of the state of cell
and of all adjacent cells:
is the state of cell
.
is the state of the cell to the west.
is the state of the cell to the south.
is the state of the cell to the east.
is the state of the cell to the north.
- Then, Pulibot determines the instruction
which corresponds to the recognized state array.
- Finally, Pulibot performs that instruction: it sets the color of cell
to color
and then it performs action
, which is one of the following actions:
- stay at cell
;
- move to one of the
adjacent cells;
- terminate the program.
- stay at cell
For example, consider the scenario displayed on the left of the following figure. Pulibot is currently
at cell with the color
. Pulibot recognizes the state array
. Pulibot may
have a program which, upon recognizing this array, sets the color of the current cell to
and
then moves to the east, as displayed in the middle and on the right of the figure:
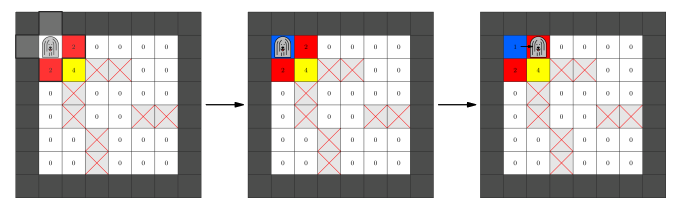
Robot Contest Rules
- At the start, Pulibot is placed at cell
and begins to execute its program.
- Pulibot is not allowed to move to a cell which is not empty.
- Pulibot's program must terminate after at most
steps.
- After the termination of Pulibot's program, empty cells in the maze should be colored such that:
- There exists a shortest path from
to
for which the color of each cell included in the path is
.
- All other empty cells have color
.
- There exists a shortest path from
- Pulibot may terminate its program at any empty cell.
For example, the following figure shows a possible maze with . The starting
configuration is displayed on the left and one acceptable coloring of empty cells after termination
is displayed on the right:
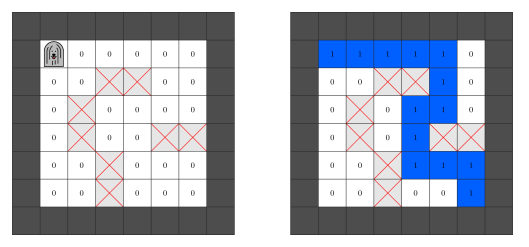
Implementation Details
You should implement the following procedure.
void program_pulibot()
- This procedure should produce Pulibot's program. This program should work correctly for all values of
and
and any maze which meets the task constraints.
- This procedure is called exactly once for each test case.
This procedure can make calls to the following procedure to produce Pulibot's program:
void set_instruction(int[] S, int Z, char A)
: array of length 5 describing a state array.
: a nonnegative integer representing a color.
: a single character representing an action of Pulibot as follows:
: stay;
: move to the west;
: move to the south;
: move to the east;
: move to the north;
: terminate the program.
- Calling this procedure instructs Pulibot that upon recognizing the state array
it should perform the instruction
.
Сalling this procedure multiple times with the same state array will result in an
Output isn't correct
verdict.
It is not required to call set_instruction
with each possible state array . However, if Pulibot
later recognizes a state array for which an instruction was not set, you will get an
Output isn't correct
verdict.
After program_pulibot
completes, the grader invokes Pulibot's program over one or more
mazes. These invocations do not count towards the time limit for your solution. The grader is not
adaptive, that is, the set of mazes is predefined in each test case.
If Pulibot violates any of the Robot Contest Rules before terminating its program, you will get an
Output isn't correct
verdict.
Example
The procedure program_pulibot
may make calls to set_instruction as follows:
Call | Instruction for State Array |
---|---|
set_instruction([0, -2, -1, 0, -2], 1, E) | Set color to |
set_instruction([0, 1, -1, 0, -2], 1, E) | Set color to |
set_instruction([0, 1, 0, -2, -2], 1, S) | Set color to |
set_instruction([0, -1, -2, -2, 1], 1, T) | Set color to |
Consider a scenario where and
, and the maze is displayed in the following figure.
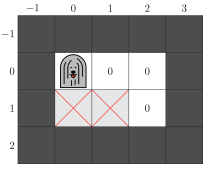
For this particular maze Pulibot's program runs in four steps. The state arrays Pulibot recognizes
and the instructions it performs correspond exactly to the four calls to set_instruction
made
above, in order. The last of these instructions terminates the program.
The following figure shows the maze before each of the four steps and the final colors after termination.
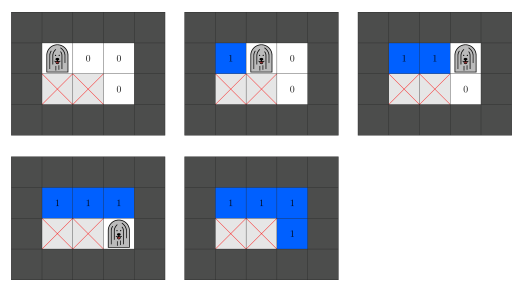
However, do note that this program of 4 instructions might not find a shortest path in other valid
mazes. Therefore, if submitted, it will receive an Output isn't correct
verdict.
Constraints
. Hence, Pulibot can use colors from
to
, inclusive.
For each maze used to test Pulibot:
- There is at least one path from cell
to cell
Subtasks
- (6 points) There is no obstacle cell in the maze.
- (10 points)
- (18 points) There is exactly one path between each pair of empty cells.
- (20 points) Each shortest path from cell
to cell
has length
.
- (46 points) No additional constraints.
If, in any of the test cases, the calls to the procedure set_instruction
or Pulibot's program over
its execution do not conform to the constraints described in Implementation Details, the score of
your solution for that subtask will be .
In each subtask, you can obtain a partial score by producing a coloring that is almost correct. Formally:
- The solution of a test case is complete if the final coloring of the empty cells satisfies Robot Contest Rules.
- The solution of a test case is partial if the final coloring looks as follows:
- There exists a shortest path from
to
for which the color of each cell included in the path is
.
- There is no other empty cell in the grid with color
.
- Some empty cell in the grid has a color other than
and
.
- There is no other empty cell in the grid with color
If your solution to a test case is neither complete nor partial, your score for the corresponding test case will be .
In subtasks 1-4, the score for a complete solution is and the score for a partial solution to a
test case is
of the points for its subtask.
In subtask 5, your score depends on the number of colors used in Pulibot's program. More
precisely, denote by the maximum value of
over all calls made to
set_instruction
. The
score of the test case is calculated according to the following table:
Condition | Score (complete) | Score (partial) |
---|---|---|
The score for each subtask is the minimum of the points for the test cases in the subtask.
Sample Grader
The sample grader reads the input in the following format:
- line
:
- line
:
Here, is an array of
arrays of
integers, describing the non-boundary cells of the maze.
if cell
is an empty cell and
if cell
is an obstacle cell.
The sample grader first calls program_pulibot()
. If the sample grader detects a protocol
violation, the sample grader prints Protocol Violation: <MSG>
and terminates, where <MSG>
is one of the following error messages:
Invalid array
:is not met for some
or the length of
is not
.
Invalid color
:is not met.
Invalid action
: characteris not one of
H
,W
,S
,E
,N
orT
.Same state array
:set_instruction
was called with the same arrayat least twice.
Otherwise, when program_pulibot
completes, the sample grader executes Pulibot's program in
the maze described by the input.
The sample grader produces two outputs.
First, the sample grader writes a log of Pulibot's actions to the file robot.bin
in the working
directory. This file serves as the input of the visualization tool described in the following section.
Second, if Pulibot's program does not terminate successfully, the sample grader prints one of the following error messages:
Unexpected state
: Pulibot recognized a state array whichset_instruction
was not called with.Invalid move
: performing an action resulted in Pulibot moving to a nonempty cell.Too many steps
: Pulibot performedsteps without terminating its program.
Otherwise, let be the state of cell
after Pulibot's program terminates. The sample
grader prints
lines in the following format:
- Line
:
Display Tool
The attachment package for this task contains a file named display.py
. When invoked, this
Python script displays Pulibot's actions in the maze described by the input of the sample grader.
For this, the binary file robot.bin
must be present in the working directory.
To invoke the script, execute the following command.
python3 display.py
A simple graphical interface shows up. The main features are as follows:
- You can observe the status of the full maze. The current location of Pulibot is highlighted by a rectangle.
- You can browse through the steps of Pulibot by clicking the arrow buttons or pressing their hotkeys. You can also jump to a specific step.
- The upcoming step in Pulibot's program is shown at the bottom. It shows the current state array and the instruction it will perform. After the final step, it shows either one of the error messages of the grader, or
Terminated
if the program successfully terminates. - To each number that represents a color, you can assign a visual background color, as well as a display text. The display text is a short string that shall appear in each cell having that color. You can assign background colors and display texts in either of the following ways:
- Set them in a dialog window after clicking on the Colors button.
- Edit the contents of the colors.txt file.
- To reload
robot.bin
, use theReload
button. It is useful if the contents ofrobot.bin
have changed.
Attachment Package
The sample grader along with sample test cases are available here.
Comments