Consider a tree consisting of vertices,
numbered from
to
.
Vertex
is called the root.
Every vertex, except for the root, has a single parent.
For every
, such that
,
the parent of vertex
is vertex
, where
.
We also assume
.
For any vertex (
),
the subtree of
is the set of the following vertices:
, and
- any vertex whose parent is
, and
- any vertex whose parent's parent is
, and
- any vertex whose parent's parent's parent is
, and
- etc.
The picture below shows an example tree consisting of vertices.
Each arrow connects a vertex to its parent,
except for the root, which has no parent.
The subtree of vertex
contains vertices
and
.
The subtree of vertex
contains all
vertices of the tree
and the subtree of vertex
contains only vertex
.
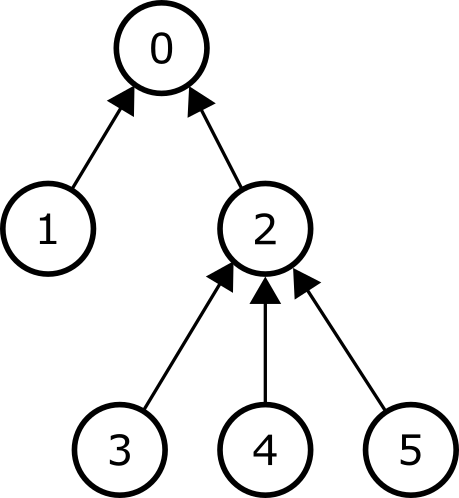
Each vertex is assigned a nonnegative integer weight.
We denote the weight of vertex (
) by
.
Your task is to write a program that will answer queries,
each specified by a pair of positive integers
.
The answer to the query should be computed as follows.
Consider assigning an integer,
called a coefficient, to each vertex of the tree.
Such an assignment is described by a sequence ,
where
(
) is the coefficient assigned to vertex
.
Let us call this sequence a coefficient sequence.
Note that the elements of the coefficient sequence can be negative,
, or positive.
For a query ,
a coefficient sequence is called valid
if, for every vertex
(
),
the following condition holds:
the sum of the coefficients of the vertices in the subtree of vertex
is not less than
and not greater than
.
For a given coefficient sequence ,
the cost of a vertex
is
,
where
denotes the absolute value of
.
Finally, the total cost is the sum of the costs of all vertices.
Your task is to compute, for each query,
the minimum total cost that can be attained by some valid coefficient sequence.
It can be shown that for any query, at least one valid coefficient sequence exists.
Implementation Details
You should implement the following two procedures:
void init(std::vector<int> P, std::vector<int> W)
,
: arrays of integers of length
specifying the parents and the weights.
- This procedure is called exactly once in the beginning of the interaction between the grader and your program in each test case.
long long query(int L, int R)
,
: integers describing a query.
- This procedure is called
times after the invocation of
init
in each test case. - This procedure should return the answer to the given query.
Constraints
for each
such that
for each
such that
in each query
Subtasks
Subtask | Score | Additional Constraints |
---|---|---|
1 | ||
2 | ||
3 | ||
4 | ||
5 | ||
6 | ||
7 | No additional constraints. |
Examples
Consider the following calls:
init([-1, 0, 0], [1, 1, 1])
The tree consists of vertices, the root and its
children.
All vertices have weight
.
query(1, 1)
In this query ,
which means the sum of coefficients in every subtree must be equal to
.
Consider the coefficient sequence
.
The tree and the corresponding coefficients (in shaded rectangles) are illustrated below.
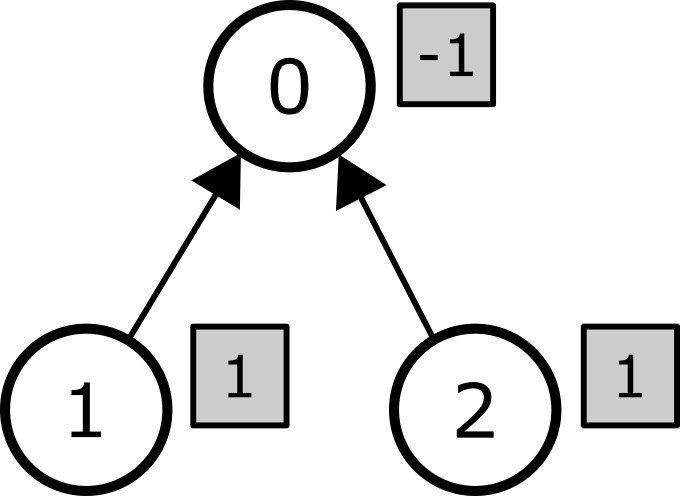
For every vertex (
), the sum of the coefficients of all vertices
in the subtree of
is equal to
.
Hence, this coefficient sequence is valid.
The total cost is computed as follows:
Vertex | Weight | Coefficient | Cost |
---|---|---|---|
0 | 1 | -1 | |
1 | 1 | 1 | |
2 | 1 | 1 |
Therefore the total cost is .
This is the only valid coefficient sequence,
therefore this call should return
.
query(1, 2)
The minimum total cost for this query is ,
and is attained when the coefficient sequence is
.
Sample Grader
Input format:
N
P[1] P[2] ... P[N-1]
W[0] W[1] ... W[N-2] W[N-1]
Q
L[0] R[0]
L[1] R[1]
...
L[Q-1] R[Q-1]
where and
(for
)
are the input arguments in the
-th call to
query
.
Note that the second line of the input contains only integers,
as the sample grader does not read the value of
.
Output format:
A[0]
A[1]
...
A[Q-1]
where
(for
)
is the value returned by the
-th call to
query
.
Attachment Package
The sample grader along with sample test cases are available here.
Comments