The Great Sphinx has a riddle for you.
You are given a graph on vertices.
The vertices are numbered from
to
.
There are
edges in the graph, numbered from
to
.
Each edge connects a pair of distinct vertices and is bidirectional.
Specifically, for each
from
to
(inclusive)
edge
connects vertices
and
.
There is at most one edge connecting any pair of vertices.
Two vertices are called adjacent
if they are connected by an edge.
A sequence of vertices (for
)
is called a path
if each two consecutive vertices
and
(for each
such that
)
are adjacent.
We say that a path
connects vertices
and
.
In the graph given to you, each pair of vertices is connected by some path.
There are colours, numbered from
to
.
Colour
is special and is called the Sphinx's colour.
Each vertex is assigned a colour.
Specifically, vertex
(
) has colour
.
Multiple vertices may have the same colour,
and there might be colours not assigned to any vertex.
No vertex has the Sphinx's colour,
that is,
(
).
A path (for
)
is called monochromatic
if
all of its vertices have the same colour,
i.e.
(for each
such that
).
Additionally, we say that vertices
and
(
,
)
are in the same monochromatic component
if and only if they are connected by a monochromatic path.
You know the vertices and edges, but you do not know which colour each vertex has. You want to find out the colours of the vertices, by performing recolouring experiments.
In a recolouring experiment,
you may recolour arbitrarily many vertices.
Specifically, to perform a recolouring experiment
you first choose an array of size
,
where for each
(
),
is between
and
inclusive.
Then, the colour of each vertex
becomes
, where the value of
is:
, that is, the original colour of
, if
, or
, otherwise.
Note that this means that you can use the Sphinx's colour in your recolouring.
Finally, the Great Sphinx announces
the number of monochromatic components in the graph,
after setting the colour of each vertex to
(
).
The new colouring is applied only for this particular recolouring experiment,
so the colours of all vertices return to the original ones after the experiment finishes.
Your task is to identify the colours of the vertices in the graph
by performing at most recolouring experiments.
You may also receive a partial score
if you correctly determine for every pair of adjacent vertices,
whether they have the same colour.
Implementation Details
You should implement the following procedure.
std::vector<int> find_colours(int N,
std::vector<int> X, std::vector<int> Y)
: the number of vertices in the graph.
,
: arrays of length
describing the edges.
- This procedure should return an array
of length
, representing the colours of vertices in the graph.
- This procedure is called exactly once for each test case.
The above procedure can make calls to the following procedure to perform recolouring experiments:
int perform_experiment(std::vector<int> E)
: an array of length
specifying how vertices should be recoloured.
- This procedure returns the number of monochromatic components
after recolouring the vertices according to
.
- This procedure can be called at most
times.
The grader is not adaptive, that is,
the colours of the vertices are fixed before a call to find_colours
is made.
Constraints
for each
such that
.
or
for each
and
such that
.
- Each pair of vertices is connected by some path.
for each
such that
.
Subtasks
Subtask | Score | Additional Constraints |
---|---|---|
1 | ||
2 | ||
3 | The graph is a path: |
|
4 | The graph is complete: |
|
5 | No additional constraints. |
In each subtask, you can obtain a partial score if your program determines correctly for every pair of adjacent vertices whether they have the same colour.
More precisely,
you get the whole score of a subtask
if in all of its test cases,
the array returned by
find_colours
is exactly the same as array
(i.e.
for all
such that
).
Otherwise,
you get
of the score for a subtask
if the following conditions hold
in all of its test cases:
for each
such that
;
- For each
such that
:
if and only if
.
Example
Consider the following call.
find_colours(4, [0, 1, 0, 0], [1, 2, 2, 3])
For this example, suppose that
the (hidden) colours of the vertices are given by
.
This scenario is shown in the following figure.
Colours are additionally represented by numbers on white labels attached to each vertex.
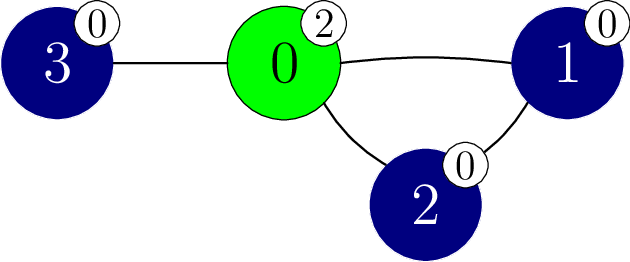
The procedure may call perform_experiment
as follows.
perform_experiment([-1, -1, -1, -1])
In this call, no vertex is recoloured, as all vertices keep their original colours.
Consider vertex and vertex
.
They both have colour
and the path
is a monochromatic path.
As a result, vertices
and
are in the same monochromatic component.
Consider vertex and vertex
.
Even though both of them have colour
,
they are in different monochromatic components
as there is no monochromatic path connecting them.
Overall, there are monochromatic components,
with vertices
,
, and
.
Thus, this call returns
.
Now the procedure may call perform_experiment
as follows.
perform_experiment([0, -1, -1, -1])
In this call, only vertex is recoloured to colour
,
which results in the colouring shown in the following figure.
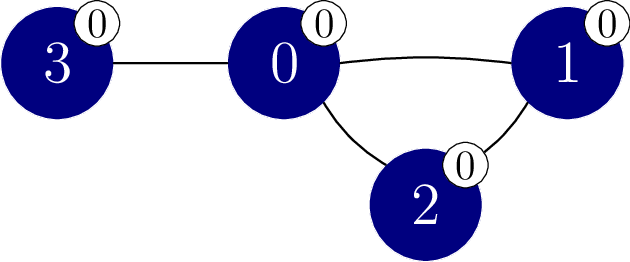
This call returns , as all the vertices belong to the same monochromatic component.
We can now deduce that vertices
,
, and
have colour
.
The procedure may then call perform_experiment
as follows.
perform_experiment([-1, -1, -1, 2])
In this call, vertex is recoloured to colour
,
which results in the colouring shown in the following figure.
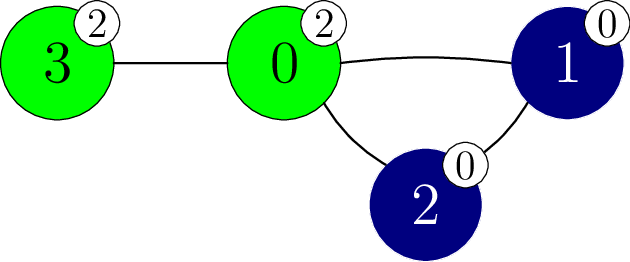
This call returns , as there are
monochromatic components,
with vertices
and
respectively.
We can deduce that vertex
has colour
.
The procedure find_colours
then returns the array .
Since
, full score is given.
Note that there are also multiple return values, for which of the score would be given, for example
or
.
Sample Grader
Input format:
N M
C[0] C[1] ... C[N-1]
X[0] Y[0]
X[1] Y[1]
...
X[M-1] Y[M-1]
Output format:
L Q
G[0] G[1] ... G[L-1]
Here, is the length of the array
returned by
find_colours
,
and is the number of calls to
perform_experiment
.
Attachment Package
The sample grader along with sample test cases are available here.
Comments